Java Notes
BoxLayout and Boxes
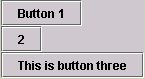
BoxLayout arranges components either horizontally or vertically in a panel. You can control alignment and spacing of the components. Complicated layouts can be made by combining many panels, some with horizontal layout and some with vertical layouts.

Several classes
are typically used:
javax.swing.BoxLayout
,
javax.swing.Box
, and
javax.swing.Box.Filler
.
To Create a JPanel with BoxLayout
Choose either a
horizontal layout (BoxLayout.X_AXIS
) or
vertical layout (BoxLayout.Y_AXIS
) for a JPanel.
JPanel p = new JPanel();
p.setLayout(new BoxLayout(p, BoxLayout.Y_AXIS));
p.add(some_component);
Unlike other layouts, the panel/container must be passed to the BoxLayout constructor.
Example
The above examples were created with this code, using either X_AXIS or Y_AXIS.
content.setLayout(new BoxLayout(content, BoxLayout.X_AXIS));
content.add(new JButton("Button 1"));
content.add(new JButton("2"));
content.add(new JButton("This is button three"));
The Box class
The Box
class was designed to be a simple, and slightly more efficient, substitute for
a JPanel
with a BoxLayout
.
Because it doesn't support everything that JPanel
does
(eg, borders), I recommend using a JPanel
with
a BoxLayout
rather than Box
.
However, the Box
class has a number of necessary
methods for working with BoxLayouts
.
Because Box
is a Container
with
BoxLayout
, all discussions of spacing and alignment
apply equally well to both JPanels with BoxLayouts and Boxes.
Creating Boxes
You can create the two kinds of boxes with:
import javax.swing.*; . . . Box vb = Box.createVerticalBox(); Box hb = Box.createHorizontalBox();
No Borders on Boxes
Boxes are lighter weight (ie, more efficient) than JPanel, but they don't support Borders. If you need borders, either use a JPanel with BoxLayout, or put the Box into a JPanel with a border.