Strings and StringBuilder
About Strings
Object A String is an object made by the String class. You can tell because when you make it is it always capitalized. It is not a primitive data type. There are 8 primitive data types -- do you remember what they are?
When you make a string, you write String s = new String("hello there");
This looks exactly like making any other type of object using the new
operator and a constructor.
Why then don't we make strings like this? Because Java kindly allows us to use a shortcut -- only for this particular type of object.
Java pretends that a String can be a primitive data type only as far as initializing it.
We can write String s = "hello there";
Methods Because String is an object it can have methods.
You can write
int len;
len = s.length();
Note that primitive data types do not have methods because they are not objects in a class.
You can never write
int x = 52;
System.out.println(x.toString());
or
System.out.println(x.length());
You can also combine methods -- because of how the . operator works. It is left associative.
if ( s1.substring(0,3).equals("pre") ) { ... }
Counting How letters are counted in String
A string is drawn as a character array in order to visualize it. BUT A string is not a character array. (You can't access letters by using s[i])
There are ways to convert arrays of characters to strings and vice versa.
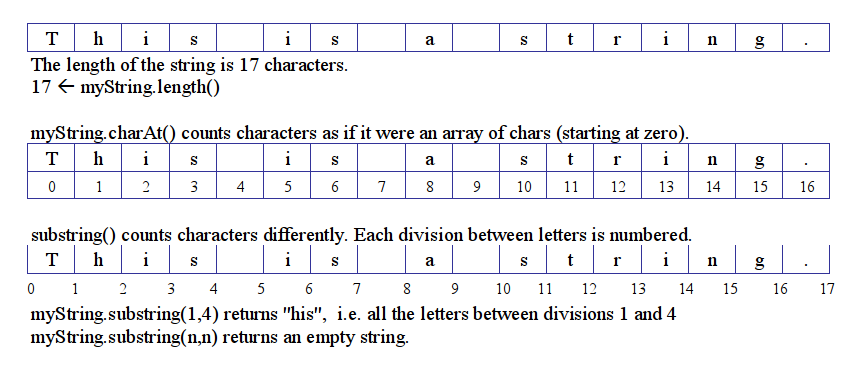
Immutable A string is immutable
A string cannot be changed. If you make a string String str = "true";
and then change it to
str = str + " or false";
you have not changed the original string.
What happens is that Java creates a new string in a different part of memory with the contents "true or false", then the variable str is changed to point to
the new place in memory and the old string gets deleted.
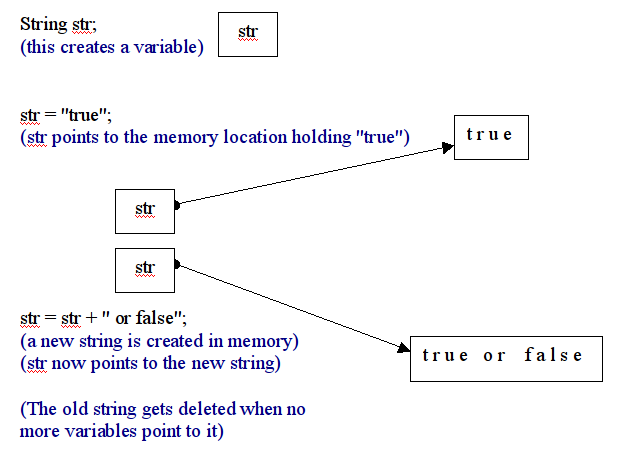
You'll notice that there are no methods in the String class that change the actual string. There is no .append(), .delete(), ... etc.
What about replace? It returns a NEW string with the characters replaced.
Why are Strings this way? I don't know, but I'm sure that there's a good reason.
Example:
String s = "hello";
s.toUpperCase(); //this will not change 's'
System.out.print(s); //prints 'hello'
You have to reassign the output of the the string method to a new (or old) variable
s = s.toUpperCase();
System.out.print(s); //prints 'HELLO'
in order to change 's'.
Problem: let's say that you have an 800 character string and want to do
for (int i =0; i < 1000; i++) { str = str + "x"; }This ends up making 1000 copies of the large string in memory! Very inefficient, slow. This is why we use StringBuilder ....
StringBuilder
StringBuilder is an object that is similar to an array of chars but a whole lot more convenient.
It is like a string that can be modified -- things can be added, deleted, and changed.
However, a StringBuilder is not identical to a String object, so there are something which you cannot do with it.
You may need to convert it back to a String first.
If you are modifying lots and lots of strings or modifying really long strings, use a StringBuilder.